Factory Pattern in .Net with an example
During my learning of design patterns, I came to know most frequent term Factory Pattern. I searched the Internet and came across numerous learning points. After a lot of search and study, I observed that to find the definition of Factory pattern.
In this article I try to explore this pattern in an easiest way with a very interesting topic Mobile.
An important aspect of software design is the manner in which objects are created. Thus, it is not only important what an object does or what it models, but also in what manner it was created.
At very first stage of an object creation is using “new” keyword, such a basic mechanism of object creation could result in design problems or added complexity to the design. On each Object creation we have to use new keyword. Factory helps you to reduce this practice and use the common interface to create an object.
The Factory Pattern is a Creational Pattern that simplifies object creation. You need not worry about the object creation; you just need to supply an appropriate parameter and factory to give you a product as needed.
· Creating objects without exposing the instantiation logic to client
· Referring to the newly created objects through a common interface
One of the most traditional designs of Factory Pattern is depicted below:
Let’s Move further and take a very general example for this situation. Which is about to get the Mobile details, wherein a customer may wish to get details of different kinds of mobile and their OS types. An illustrative image is depict below:
A mobile detail provides three different types of mobiles (Samsung, Apple and Nokia). Based on the client choice, an application provides the details of specific mobile. The easiest way to get the details is, client applications will instantiate the desired class by directly using the
However It shows the tight coupling between Client and the Mobile class. Client application has to be aware of all the concrete Mobiles (Samsung, Apple and Nokia) and their instantiation logic. This methodology often introduces a great deal of the rigidity in the system. Also the coupling introduced between classes is extremely difficult to overcome if the requirements change on frequent interval.
Factory does the same work in bit different manner to create an object. Which is not visible to client? How Factory overcome on these fall backs by delegating the task of object creation to a factory-class (e.g. FactoryClass.cs) in our sample. The factory-class completely abstracts the creation and initialization of the product from the client-application. It helps to the client application to keep away from Object creation and the concrete details of mobile.
A typical image of a Factory pattern is depicted below to understand the fact.
The above given images simply tells the association of Client app with Factory class and the creation of object Via Factory class.
We do have a Factory class named as Factory Class, Factory class is responsible to create an object and its initiation .Factory class has one method named as CreateMobileObject which creates an object on behalf of the parameter being passed by user (client) and returns mobile type.
Code snippet for Factory class:
Code snippet for the Main is as follows:

Press F5 and you will get the result like the below black window:

The above image gives you the details of two mobiles which we passed into factory class method.
· Easy to implement
· Client application code doesn’t have to change drastically
· Moreover, the tight coupling between client and mobile classes is overcome and turned into coupling between factory and mobile classes. Hence client need not know the instantiation logic of products.
· If we add any new product (mobile), we need a new case statement in CreateMobileObject method of Factory class. This violates open/closed design principle.
· We can avoid modifying the Factory class by using sub classing. But sub classing means replacing all the factory class references everywhere through the code.
· We have tight coupling between Factory class and products.
·
Sample App is attached as an reference.
Thanks,
Keep Coding and Be Happy
During my learning of design patterns, I came to know most frequent term Factory Pattern. I searched the Internet and came across numerous learning points. After a lot of search and study, I observed that to find the definition of Factory pattern.
In this article I try to explore this pattern in an easiest way with a very interesting topic Mobile.
An important aspect of software design is the manner in which objects are created. Thus, it is not only important what an object does or what it models, but also in what manner it was created.
At very first stage of an object creation is using “new” keyword, such a basic mechanism of object creation could result in design problems or added complexity to the design. On each Object creation we have to use new keyword. Factory helps you to reduce this practice and use the common interface to create an object.
Factory Pattern Definition
GOF says: Define an interface for creating an object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses.The Factory Pattern is a Creational Pattern that simplifies object creation. You need not worry about the object creation; you just need to supply an appropriate parameter and factory to give you a product as needed.
· Creating objects without exposing the instantiation logic to client
· Referring to the newly created objects through a common interface
One of the most traditional designs of Factory Pattern is depicted below:
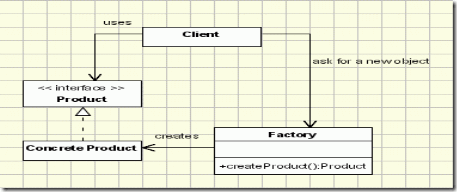
Let’s Move further and take a very general example for this situation. Which is about to get the Mobile details, wherein a customer may wish to get details of different kinds of mobile and their OS types. An illustrative image is depict below:
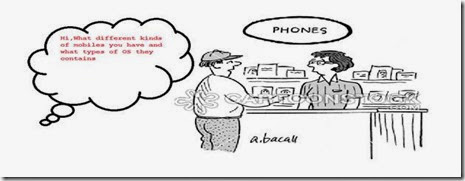
A mobile detail provides three different types of mobiles (Samsung, Apple and Nokia). Based on the client choice, an application provides the details of specific mobile. The easiest way to get the details is, client applications will instantiate the desired class by directly using the
new
keyword. However It shows the tight coupling between Client and the Mobile class. Client application has to be aware of all the concrete Mobiles (Samsung, Apple and Nokia) and their instantiation logic. This methodology often introduces a great deal of the rigidity in the system. Also the coupling introduced between classes is extremely difficult to overcome if the requirements change on frequent interval.
Factory does the same work in bit different manner to create an object. Which is not visible to client? How Factory overcome on these fall backs by delegating the task of object creation to a factory-class (e.g. FactoryClass.cs) in our sample. The factory-class completely abstracts the creation and initialization of the product from the client-application. It helps to the client application to keep away from Object creation and the concrete details of mobile.
A typical image of a Factory pattern is depicted below to understand the fact.

The above given images simply tells the association of Client app with Factory class and the creation of object Via Factory class.
We do have a Factory class named as Factory Class, Factory class is responsible to create an object and its initiation .Factory class has one method named as CreateMobileObject which creates an object on behalf of the parameter being passed by user (client) and returns mobile type.
Code snippet for Factory class:
There are few classes which I’ve built for mobile classes which implements IMobile interface.1: public static class FactoryClass2: {3: public static IMobile CreateMobileObject(MobileType mobileType)4: {5: IMobile objIMobile = null;6: switch (mobileType)7: {8: case MobileType.Samsung:
9: objIMobile = new Samsung();
10: return objIMobile;
11:12: case MobileType.Apple:
13: objIMobile = new Apple();
14: return objIMobile;
15:16: case MobileType.Nokia:
17: objIMobile = new Nokia();
18: return objIMobile;
19:20: default:
21: return null;
22:23: }24: }25: }
Now moving further and pass a parameter to the static method CreateMobileObject() accepts a parameter of desired type.1: public class Samsung : IMobile2: {3: public string ModelName()4: {5: return "Samsung Galaxy Grand";6: }7:8: public string OperatingSystem()9:10: { return "Samsung Uses Android OS For Galaxy Mobile series "; }11:12: }13:14: public class Apple : IMobile15: {16: public string ModelName()17: {18: return "Apple IPhone 5";19: }20:21: public string OperatingSystem()22:23: { return "Apple Uses ios OS for Apple Mobiles "; }24:25: }26:27: public class Nokia : IMobile28: {29: public string ModelName()30: {31: return "Nokia Lumia 960";32: }33:34: public string OperatingSystem()35:36: { return "Nokia Uses Symbion OS for Lumia Mobile series "; }37:38: }
Code snippet for the Main is as follows:

Press F5 and you will get the result like the below black window:
The above image gives you the details of two mobiles which we passed into factory class method.
Advantages
· Easy to implement
· Client application code doesn’t have to change drastically
· Moreover, the tight coupling between client and mobile classes is overcome and turned into coupling between factory and mobile classes. Hence client need not know the instantiation logic of products.
Disadvantages
· If we add any new product (mobile), we need a new case statement in CreateMobileObject method of Factory class. This violates open/closed design principle.
· We can avoid modifying the Factory class by using sub classing. But sub classing means replacing all the factory class references everywhere through the code.
· We have tight coupling between Factory class and products.
·
Sample App is attached as an reference.
Thanks,
Keep Coding and Be Happy

Hi Sachin,
ReplyDeleteGreat learning, just a little suggestion to enhance your code for better readability, you can instead of returning the object in each case statement in your Factory Class, just create the object in each case and then return it finally once in the last.
Anyhow, there's only one case statement that would be executed, so no need to return from each case.
Considered your thoughts.Thanks For reading this article :)
ReplyDelete