Database Table Encryption Using Symmetric Key in SQL Server 2008 R2
The purpose of this article is to provide security to a database column's value so that no one can understand what the actual value is.

Scenario: Actually a few days ago I had the situation to add one more layer of security, like encryption and decryption of some keys (for example SaltKey or RSAKey), that were being used in my C# code base file. These keys were being used to encrypt and decrypt the username and password, however I don't want to make these keys public to anyone (for example SaltKey or RSAKey) , because anyone can read this easily using reflector if obfuscation is not applied to the C# code base file (.cs file). So I thought to put these keys into the database and put up all the columns values in encrypted mode and decrypt it since I needed the actual values of these keys.
I studied about this from various portals before implementing. In this article I'll also share some findings that may be helpful to you to create rudimentary queries.
There are many approaches to implement encryption but I've chosen column encryption of a database table. I just encrypted and decrypted the data placed in the table. This approach takes little time to do the required procedure than the other approach.
So let's start with encryption and decryption process executed with a Symmetric Key. I've created a database with the name DBEncrypt with a table “TestTable” that has three columns in it. Kindly look at the screenshot given below:
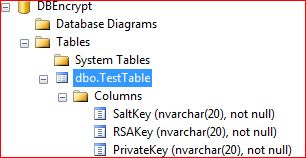
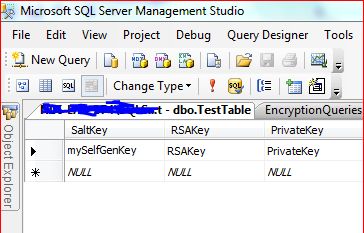
If you notice in the image shown above there are three columns with some text value. My main objective is to encrypt those values and decrypt accordingly.
Create a master key, each database can have one master key and this master key used to protect the private keys of the certificates. After this we'll create a certificate to encrypt the data in the database and symmetric key (the symmetric key used by the sender and the receiver of a message, it's a common key used to encrypt and decrypt the message). We've created a symmetric key using the certificate (EncryptTestCert).
1: CREATE MASTER KEY ENCRYPTION
2: BY PASSWORD = 'Support@123'
3:
4: CREATE CERTIFICATE EncryptTestCert
5: WITH SUBJECT = 'SupportCert'
6:
7: CREATE SYMMETRIC KEY TestTableKey
8: WITH ALGORITHM = TRIPLE_DES ENCRYPTION
9: BY CERTIFICATE EncryptTestCert.
Now it's time to create another three columns that will keep encrypted data of these three columns (SaltKey, RSAKey and PrivateKey) of table respectively. I've also added one more Column SaltKeyEnCol2 as nvarchar(max). I will share the reason to create an extra column later in this document.
The code given below encrypts three columns (SaltKey, RSAKey and PrivateKey) values to the newly created
1: ALTER TABLE TestTable
2: ADD SaltKeyEnCol VARBINARY(256),RSAKeyEnCol VARBINARY(256),PrivateKeyEnCol VARBINARY(256)
3:
4: ALTER TABLE TestTable
5: ADD SaltKeyEnCol2 nvarchar(max)
6:
columns above with an alter commnad. Also note that we are using the same certificate(EncryptTestCert) to open the symmetric key and make it available for use. Kindly have a look at the syntax given below.
1: OPEN SYMMETRIC KEY TestTableKey DECRYPTION
2: BY CERTIFICATE EncryptTestCert,
3: UPDATE TestTable
4: SET SaltKeyEnCol = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),SaltKey),RSAKeyEnCol = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),RSAKey),PrivateKeyEnCol = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),PrivateKey)
5:
Kindly manually close the symmetric key otherwise it will remain open for the current session.
After the execution of the preceding query you can have a look and see the affects made into the database. Kindly have a look at the image given below:
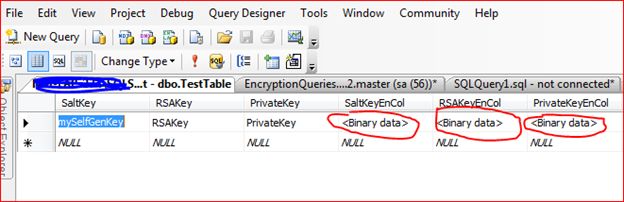
If you notice in the image above, it only shows you <Binary data> in encrypted Columns. Which was a little tricky for me initially, after looking at these values I thought I made some mistake and the data doesn't look OK.
To cater this issue, I again created another column with the name “SaltKeyEnCol2” as I have shared this in the preceding in the document and is also shown below. It helped me to see the actual value.
- ALTER TABLE TestTable
- ADD SaltKeyEnCol2 nvarchar(max)
Please execute the SQL query given below and see the effect.
- OPEN SYMMETRIC KEY TestTableKey DECRYPTION
- BY CERTIFICATE EncryptTestCert
- UPDATE TestTable SET SaltKeyEnCol2 = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),SaltKey)
The column SaltKeyEnCol2 has some encrypted value in SaltKeyEnCol2 as depicted below in the screen shot rather than the <Binary Data> value.

As we can see above, we've achived the encryption of the required columns. Now the turn is to decrypt the data placed in the encrypted columns. Kindly refer to the image given below with the outcome.
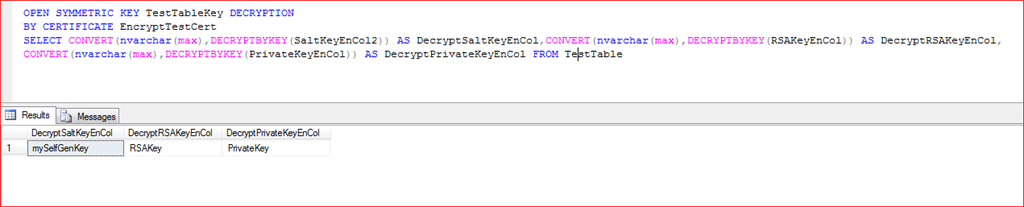
Kindly find in the following the complete database query script.
1: CREATE MASTER KEY ENCRYPTION
2: BY PASSWORD = 'Support@123'
3:
4: CREATE CERTIFICATE EncryptTestCert
5: WITH SUBJECT = 'SupportCert'
6:
7: CREATE SYMMETRIC KEY TestTableKey
8: WITH ALGORITHM = TRIPLE_DES ENCRYPTION
9: BY CERTIFICATE EncryptTestCert
10:
11: ALTER TABLE TestTable
12: ADD SaltKeyEnCol VARBINARY(256),RSAKeyEnCol VARBINARY(256),PrivateKeyEnCol VARBINARY(256)
13:
14: ALTER TABLE TestTable
15: ADD SaltKeyEnCol3 nvarchar(max)
16: --drop COLUMN SaltKeyEnCol3
17:
18:
19: OPEN SYMMETRIC KEY TestTableKey DECRYPTION
20: BY CERTIFICATE EncryptTestCert
21: UPDATE TestTable SET SaltKeyEnCol3 = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),SaltKey)
22:
23:
24: OPEN SYMMETRIC KEY TestTableKey DECRYPTION
25: BY CERTIFICATE EncryptTestCert,
26: UPDATE TestTable
27: SET SaltKeyEnCol2 = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),SaltKey),RSAKeyEnCol = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),RSAKey),PrivateKeyEnCol = ENCRYPTBYKEY(KEY_GUID('TestTableKey'),PrivateKey)
28:
29: CLOSE SYMMETRIC KEY TestTableKey;
30:
31: OPEN SYMMETRIC KEY TestTableKey DECRYPTION
32: BY CERTIFICATE EncryptTestCert
33: SELECT CONVERT(nvarchar(max),DECRYPTBYKEY(SaltKeyEnCol2)) AS DecryptSaltKeyEnCol FROM TestTable
34:
35: OPEN SYMMETRIC KEY TestTableKey DECRYPTION
36: BY CERTIFICATE EncryptTestCert
37: SELECT CONVERT(nvarchar(max),DECRYPTBYKEY(SaltKeyEnCol2)) AS DecryptSaltKeyEnCol,CONVERT(nvarchar(max),DECRYPTBYKEY(RSAKeyEnCol)) AS DecryptRSAKeyEnCol,
38: CONVERT(nvarchar(max),DECRYPTBYKEY(PrivateKeyEnCol)) AS DecryptPrivateKeyEnCol FROM TestTable
39:
40: CLOSE SYMMETRIC KEY TestTableKey
I hope it will help you somewhere. I took the idea from: Introduction to SQL Server Encryption and Symmetric Key Encryption.
Thanks For reading this article
To learn more about MVC please go to the following link.
MVC Articles
Thanks.
Keep coding and Stay Happy