Let Vs Into Keywords in LINQ
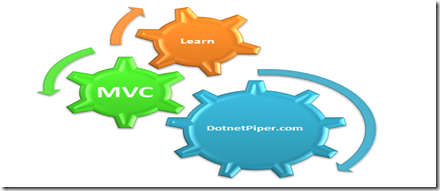
This article shows two keywords of LINQ. These keywords are very helpful when working with a set of object collections using LINQ.
First I will go through the Into keyword and its use.
I used the following collection in this article, on which I've done actions in this article.
1: List<Mobile> objMobileCollection = new List<Mobile>
2: {3: new Mobile{Provider="Apple",ModelName="IPhone3",ModelNumber="I100",MemoryCard=4},4: new Mobile{Provider="Apple",ModelName="IPhone4",ModelNumber="I101",MemoryCard=8},5: new Mobile{Provider="Apple",ModelName="IPhone4S",ModelNumber="I102",MemoryCard=16},6: new Mobile{Provider="Apple",ModelName="IPhone5",ModelNumber="I103",MemoryCard=32},7: new Mobile{Provider="Samsung",ModelName="GalaxyS",ModelNumber="S001",MemoryCard=4},8: new Mobile{Provider="Samsung",ModelName="GalaxyS2",ModelNumber="S002",MemoryCard=16},9: new Mobile{Provider="Samsung",ModelName="GalaxyS3",ModelNumber="S003",MemoryCard=20},10: new Mobile{Provider="Samsung",ModelName="Grand",ModelNumber="G001",MemoryCard=4},11: new Mobile{Provider="Nokia",ModelName="LUMIA530",ModelNumber="N1",MemoryCard=8},12: new Mobile{Provider="Nokia",ModelName="LUMIA730",ModelNumber="N2",MemoryCard=16},13: new Mobile{Provider="Nokia",ModelName="LUMIA930",ModelNumber="N3",MemoryCard=20},14: };
Into keyword
The Into keyword allows creating a temporary variable to store the results of a group, join or select clause into a new variable.
1: var resultSet = from mobile in objMobileCollection
2: group mobile by new { mobile.Provider } into mGroup
3: orderby mGroup.Key.Provider4: select new5: {6: Provider = mGroup.Key.Provider,7: ModelName = mGroup.OrderBy(x => x.ModelName),8: //ModelNumber = mGroup.OrderBy(x => x.ModelNumber),9: //MemoryCard = mGroup.OrderBy(x => x.MemoryCard),10: };11:
In the above query after applying Into on mobile groping it creates the type mGroup variable to apply the next filter or create a temporary variable “mGroup” for further operations.
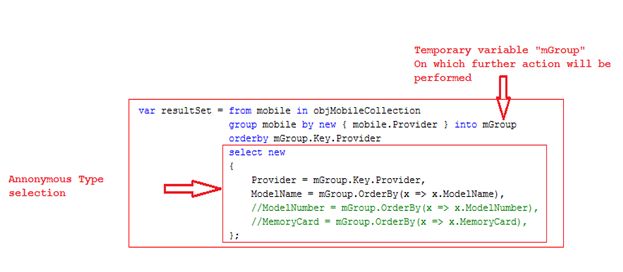
As in the code below:
1: foreach (var items in resultSet)
2: {3: Console.WriteLine("{0} - {1}", items.Provider, items.ModelName.Count());
4: Console.WriteLine("------------------------------------------------");
5: foreach (var item in items.ModelName)
6: {7: Console.WriteLine(item.Provider + "\t" + item.ModelName + "\t\t" + item.ModelNumber.Trim() + "\t" + item.MemoryCard);8: }9: Console.WriteLine();10: }11: Console.ReadLine();12:
Please have a look at the output as shown in the following image:
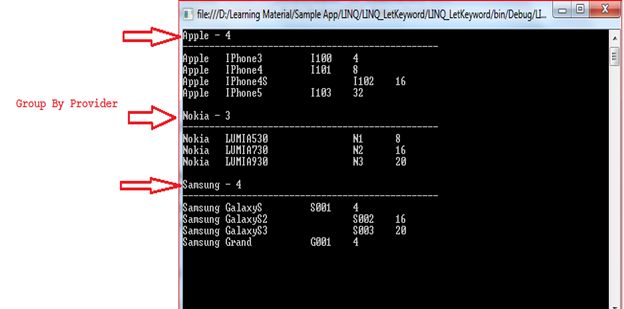
Let Keyword
The Let keyword permits you to store the result of the query that can be used further in subsequent queries.
It creates a new variable and initializes it with the result of the expression and can be used further in queries just the opposite of the Into keyword, which means you created a new variable and you also can use the previous variable so you can use both in the further operations.
1: var resultSetLet = (from mobile in objMobileCollection
2: group mobile by new { mobile.Provider } into mGroup
3: //orderby mGroup.Key.Provider,mGroup.Key.MemoryCard4: let avgMemory = mGroup.Sum(x => x.MemoryCard) / mGroup.Count()
5: where avgMemory > 116: select new7: {8: Provider = mGroup.GroupBy(x => x.Provider),9: ModelName = mGroup.OrderBy(m => m.ModelName),10: ModelNumber = mGroup.OrderBy(x => x.ModelNumber)11: //MemoryCard = mGroup.OrderBy(x => x.MemoryCard),12: }).ToList();13:
Kindly refer to the image shown below for further reference:
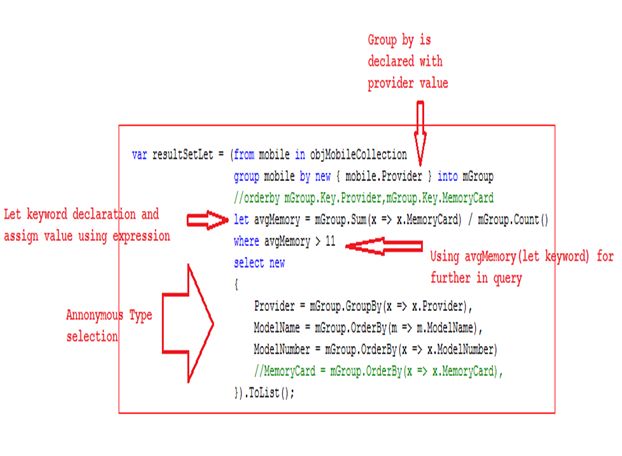
Please have a look at the complete code shown below:
1: var resultSetLet = (from mobile in objMobileCollection
2: group mobile by new { mobile.Provider } into mGroup
3: //orderby mGroup.Key.Provider,mGroup.Key.MemoryCard4: let avgMemory = mGroup.Sum(x => x.MemoryCard) / mGroup.Count()
5: where avgMemory > 116: select new7: {8: Provider = mGroup.GroupBy(x => x.Provider),9: ModelName = mGroup.OrderBy(m => m.ModelName),10: ModelNumber = mGroup.OrderBy(x => x.ModelNumber)11: //MemoryCard = mGroup.OrderBy(x => x.MemoryCard),12: }).ToList();13:14: foreach (var items in resultSetLet)
15: {16: Console.WriteLine("{0} - {1}", items.Provider, items.ModelNumber.Count());
17: Console.WriteLine("------------------------------------------------");
18: foreach (var item in items.ModelNumber)
19: {20: Console.WriteLine(item.Provider + "\t" + item.ModelName + "\t\t" + item.ModelNumber + "\t" + item.MemoryCard);21: }22: Console.WriteLine();23: }24: Console.ReadLine();
Please have a look at the output as shown below in the image:
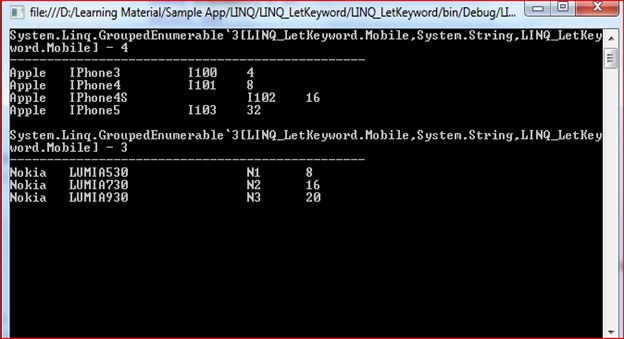
Note: Group by multiple columns in LINQ to SQL as shown in the image below:
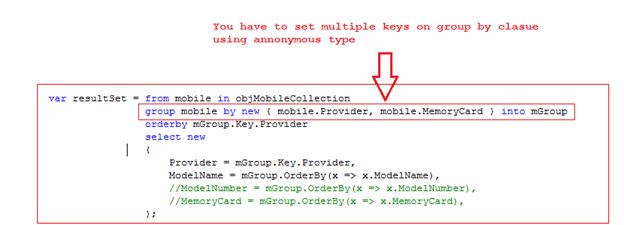
This is the complete code used in this article.
1: using System;
2: using System.Collections.Generic;
3: using System.Linq;
4: using System.Text;
5:6: namespace LINQ_LetKeyword
7: {8: #region hiddencode9: //class Employee
10: //{11: // public string Name { get; set; }12: // public string EmpID { get; set; }13: // public int Salary { get; set; }14:15: //}16: #endregion17:18: class Mobile
19: {20: public string Provider { get; set; }21: public string ModelName { get; set; }22: public string ModelNumber { get; set; }23: public int MemoryCard { get; set; }24: }25:26: class Program
27: {28: static void Main(string[] args)29: {30:31:32:33: List<Mobile> objMobileCollection = new List<Mobile> {
34: new Mobile{Provider="Apple",ModelName="IPhone3",ModelNumber="I100",MemoryCard=4},35: new Mobile{Provider="Apple",ModelName="IPhone4",ModelNumber="I101",MemoryCard=8},36: new Mobile{Provider="Apple",ModelName="IPhone4S",ModelNumber="I102",MemoryCard=16},37: new Mobile{Provider="Apple",ModelName="IPhone5",ModelNumber="I103",MemoryCard=32},38: new Mobile{Provider="Samsung",ModelName="GalaxyS",ModelNumber="S001",MemoryCard=4},39: new Mobile{Provider="Samsung",ModelName="GalaxyS2",ModelNumber="S002",MemoryCard=16},40: new Mobile{Provider="Samsung",ModelName="GalaxyS3",ModelNumber="S003",MemoryCard=20},41: new Mobile{Provider="Samsung",ModelName="Grand",ModelNumber="G001",MemoryCard=4},42: new Mobile{Provider="Nokia",ModelName="LUMIA530",ModelNumber="N1",MemoryCard=8},43: new Mobile{Provider="Nokia",ModelName="LUMIA730",ModelNumber="N2",MemoryCard=16},44: new Mobile{Provider="Nokia",ModelName="LUMIA930",ModelNumber="N3",MemoryCard=20},45:46: };47:48:49: var resultSet = from mobile in objMobileCollection
50: group mobile by new { mobile.Provider, mobile.MemoryCard } into mGroup
51: orderby mGroup.Key.Provider52: select new53: {54: Provider = mGroup.Key.Provider,55: ModelName = mGroup.OrderBy(x => x.ModelName),56: //ModelNumber = mGroup.OrderBy(x => x.ModelNumber),57: //MemoryCard = mGroup.OrderBy(x => x.MemoryCard),58: };59:60: foreach (var items in resultSet)
61: {62: Console.WriteLine("{0} - {1}", items.Provider, items.ModelName.Count());
63: Console.WriteLine("------------------------------------------------");
64: foreach (var item in items.ModelName)
65: {66: Console.WriteLine(item.Provider + "\t" + item.ModelName + "\t\t" + item.ModelNumber.Trim() + "\t" + item.MemoryCard);67: }68: Console.WriteLine();69: }70: Console.ReadLine();71:72:73: /////////////Var Keyword uses74:75:76: //var resultSetLet = (from mobile in objMobileCollection
77: // group mobile by new { mobile.Provider } into mGroup
78: // //orderby mGroup.Key.Provider,mGroup.Key.MemoryCard79: // let avgMemory = mGroup.Sum(x => x.MemoryCard) / mGroup.Count()
80: // where avgMemory > 1181: // select new82: // {83: // Provider = mGroup.GroupBy(x => x.Provider),84: // ModelName = mGroup.OrderBy(m => m.ModelName),85: // ModelNumber = mGroup.OrderBy(x => x.ModelNumber)86: // //MemoryCard = mGroup.OrderBy(x => x.MemoryCard),87: // }).ToList();88:89: //foreach (var items in resultSetLet)
90: //{91: // Console.WriteLine("{0} - {1}", items.Provider, items.ModelNumber.Count());
92: // Console.WriteLine("------------------------------------------------");
93: // foreach (var item in items.ModelNumber)
94: // {95: // Console.WriteLine(item.Provider + "\t" + item.ModelName + "\t\t" + item.ModelNumber + "\t" + item.MemoryCard);96: // }97: // Console.WriteLine();98: //}99: //Console.ReadLine();100: //var objresult = from emp in objEmployee
101: // let totalSalary = objEmployee.Sum(sal => sal.Salary)
102: // let avgSalary = totalSalary / 5
103: // where avgSalary > emp.Salary104: // select emp;
105:106:107: }108: }109: }110:
I wish it will help you utilize both features at the best.
To learn more about MVC please go to the following link.
MVC Articles
Thanks.
Enjoy coding and reading.